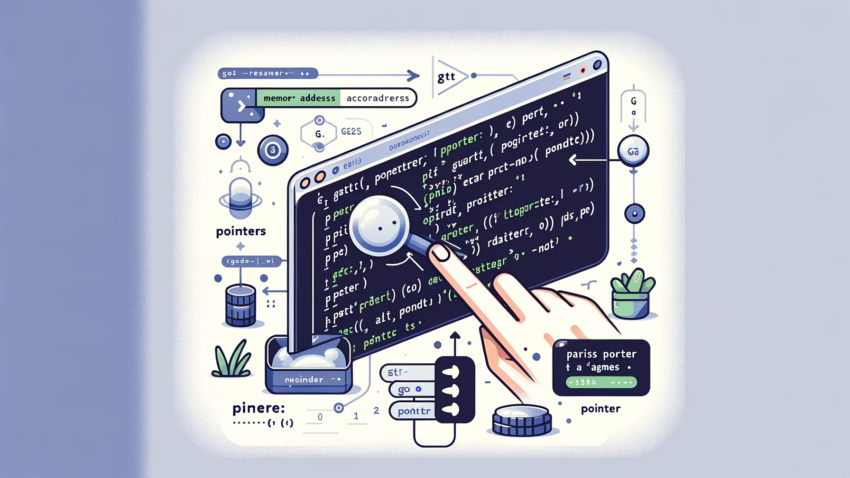
Understanding pointers in the GO language
Go, also known as Golang, is a statically typed, compiled programming language designed at Google. Known for its simplicity, efficiency, and strong support for concurrent programming, Go has become a popular choice for many developers. One of the key features of Go, borrowed from languages like C and C++, is the use of pointers. Understanding pointers is crucial for efficient memory management and optimization in Go. This essay explores the concept of pointers in Go, how they are used, and their significance in programming.
What is a pointer
In programming, a pointer is a variable that stores the memory address of another variable. Rather than containing a direct value, a pointer holds the address where the value is stored. This concept allows for more efficient and flexible data manipulation, particularly in situations where large data structures need to be passed around functions without copying their contents.
Declaring Pointers
In Go, pointers are declared using the * operator. For example, to declare a pointer to an integer, you would use *int. Here’s a basic example of declaring and using pointers in Go:
package main
import "fmt"
func main() {
var a int = 42
var p *int = &a
fmt.Println("Value of a:", a)
fmt.Println("Address of a:", &a)
fmt.Println("Value of p:", p)
fmt.Println("Value pointed to by p:", *p)
}
In this example, a is an integer variable, and p is a pointer to an integer. The & operator is used to get the address of a, which is then assigned to p. The * operator is used to dereference the pointer, i.e., to access the value stored at the address pointed to by p.
Pointer Operations
There are several operations you can perform with pointers in Go:
- Assignment: You can assign the address of a variable to a pointer.
- Dereferencing: Using the * operator, you can access or modify the value at the memory address a pointer refers to.
- Pointer Arithmetic: Unlike C and C++, Go does not support pointer arithmetic. This design choice reduces complexity and avoids common bugs related to pointer manipulation.
Passing Pointers to Functions
Passing a pointer to a function allows the function to modify the value at the pointer’s address. This is particularly useful for large data structures, as it avoids the overhead of copying data. Here’s an example:
package main
import "fmt"
func increment(p *int) {
*p++
}
func main() {
var a int = 42
fmt.Println("Before increment:", a)
increment(&a)
fmt.Println("After increment:", a)
}
In this example, the increment function takes a pointer to an integer and increments the value at that address. The &a syntax passes the address of a to the function.
Pointers and Slices
Slices in Go are a powerful data structure that provides a more flexible and convenient way to work with arrays. Under the hood, a slice is a struct that includes a pointer to an array, the length of the segment, and its capacity. This internal use of pointers makes slices efficient, as operations on slices do not require copying the underlying array data.
Pointers and Structs
Pointers are often used with structs in Go to efficiently handle and manipulate data structures. Here’s an example:
package main
import "fmt"
type Person struct {
name string
age int
}
func changeName(p *Person, newName string) {
p.name = newName
}
func main() {
person := Person{name: "Alice", age: 30}
fmt.Println("Before name change:", person)
changeName(&person, "Bob")
fmt.Println("After name change:", person)
}
In this example, the changeName function takes a pointer to a Person struct and modifies the name field. Passing a pointer to the struct allows the function to alter the original struct without copying it.
Conclusion
Pointers are a fundamental aspect of Go programming, enabling efficient memory management and data manipulation. Understanding how to declare, use, and manipulate pointers is crucial for writing performant Go code. By leveraging pointers, developers can optimize their programs, especially when dealing with large data structures or needing to pass data efficiently between functions. While Go simplifies many aspects of programming, a solid grasp of pointers is essential for any Go programmer aiming to write high-performance applications.
Leave a Reply
You must be logged in to post a comment.